Do you want to become an expert in Spring Boot CRUD operations? This comprehensive tutorial demonstrates how to use MySQL to create a Spring Boot CRUD REST API example. This guide offers a step-by-step method for creating a solid RESTful API, regardless of your level of experience.
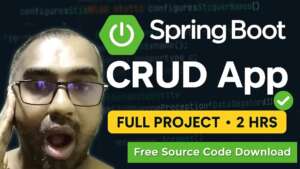
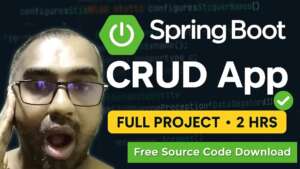
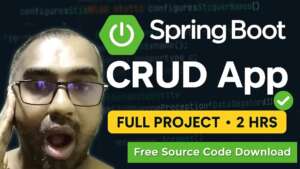
Introduction to Spring Boot and RESTful APIs
Spring Boot provides an easy-to-setup framework that facilitates the development of Java applications. When combined with RESTful APIs, it facilitates efficient client-server communication. In this lesson, we’ll focus on a Spring Boot CRUD example that demonstrates how to create, read, update, and delete data using a MySQL database.
Setting Up the Project with Spring Initializr
Begin by generating your project using Spring Initializr:
Project: Maven
Language: Java
Spring Boot: 3.4.5
Dependencies:
Spring Web
Spring Data JPA
MySQL Driver
Download the generated project and open it in your IntelliJ IDEA IDE.
Configuring the MySQL Database
In your application.properties file, set up the database connection
spring.application.name=StudentCrud server.port=8085 spring.jpa.hibernate.ddl-auto=update spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/brmschool?createDatabaseIfNotExist=true spring.datasource.username=root spring.datasource.password=root123 #jpa vendor adapter configuration spring.jpa.database-platform=org.hibernate.dialect.MySQL57Dialect spring.jpa.generate-ddl=true spring.jpa.show-sql=true
Ensure that the MySQL database brmschool exists and is accessible with the provided credentials.
Creating the Student Entity
Define the Student entity to map to the student table in your database
package com.SpringProject.StudentCrud.entity; import jakarta.persistence.*; @Entity @Table(name ="student") public class Student { @Id //primary key @GeneratedValue(strategy = GenerationType.AUTO) @Column(name="student_id", length = 45) private int studentid; @Column(name="student_name",length = 80) private String studentname; @Column(name="address",length = 100) private String address; @Column(name="mobile",length = 12) private int mobile; @Column(name="active",columnDefinition = "TINYINT default 1") private boolean active; public Student(int studentid, String studentname, String address, int mobile, boolean active) { this.studentid = studentid; this.studentname = studentname; this.address = address; this.mobile = mobile; this.active = active; } public Student() { } public Student(String studentname, String address, int mobile, boolean active) { this.studentname = studentname; this.address = address; this.mobile = mobile; this.active = active; } public int getStudentid() { return studentid; } public void setStudentid(int studentid) { this.studentid = studentid; } public String getStudentname() { return studentname; } public void setStudentname(String studentname) { this.studentname = studentname; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public int getMobile() { return mobile; } public void setMobile(int mobile) { this.mobile = mobile; } public boolean isActive() { return active; } public void setActive(boolean active) { this.active = active; } @Override public String toString() { return "Student{" + "studentid=" + studentid + ", studentname='" + studentname + '\'' + ", address='" + address + '\'' + ", mobile=" + mobile + ", active=" + active + '}'; } }
Implementing Data Transfer Objects (DTOs)
Begin by creating DTOs to pass data between different layers of the system
StudentDTO
package com.SpringProject.StudentCrud.dto; import jakarta.persistence.Column; import jakarta.persistence.GeneratedValue; import jakarta.persistence.GenerationType; import jakarta.persistence.Id; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @AllArgsConstructor @NoArgsConstructor @Data public class StudentDTO { private int studentid; private String studentname; private String address; private int mobile; private boolean active; }
StudentSaveDTO
package com.SpringProject.StudentCrud.dto; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @AllArgsConstructor @NoArgsConstructor @Data public class StudentSaveDTO { private String studentname; private String address; private int mobile; private boolean active; }
StudentUpdateDTO
package com.SpringProject.StudentCrud.dto; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @AllArgsConstructor @NoArgsConstructor @Data public class StudentUpdateDTO { private int studentid; private String studentname; private String address; private int mobile; private boolean active; }
Creating the Repository Interface
Connect to the database using Spring Data JPA.
package com.SpringProject.StudentCrud.repo; import com.SpringProject.StudentCrud.entity.Student; import org.springframework.data.jpa.repository.JpaRepository; import java.util.List; public interface StudentRepo extends JpaRepository<Student,Integer> { List<Student> findAllByStudentnameEquals(String name); }
Developing the Service Layer
Define the service interface.
package com.SpringProject.StudentCrud.service; import com.SpringProject.StudentCrud.dto.StudentDTO; import com.SpringProject.StudentCrud.dto.StudentSaveDTO; import com.SpringProject.StudentCrud.dto.StudentUpdateDTO; import java.util.List; public interface StudentService { String addStudent(StudentSaveDTO studentSaveDTO); List<StudentDTO> getAllStudent(); String updateStudent(StudentUpdateDTO studentUpdateDTO); boolean deleteStudent(int id); StudentDTO getStudentById(int id); List<StudentDTO> findStudentName(String name); }
Implement the service
package com.SpringProject.StudentCrud.service.IMPL; import com.SpringProject.StudentCrud.dto.StudentDTO; import com.SpringProject.StudentCrud.dto.StudentSaveDTO; import com.SpringProject.StudentCrud.dto.StudentUpdateDTO; import com.SpringProject.StudentCrud.entity.Student; import com.SpringProject.StudentCrud.repo.StudentRepo; import com.SpringProject.StudentCrud.service.StudentService; import org.hibernate.annotations.NotFound; import org.modelmapper.ModelMapper; import org.modelmapper.TypeToken; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.ArrayList; import java.util.List; import java.util.Optional; @Service public class StudentServiceIMPL implements StudentService { @Autowired private StudentRepo studentRepo; @Autowired private ModelMapper modelMapper; @Override public String addStudent(StudentSaveDTO studentSaveDTO) { Student student = new Student( studentSaveDTO.getStudentname(), studentSaveDTO.getAddress(), studentSaveDTO.getMobile(), studentSaveDTO.isActive() ); studentRepo.save(student); return student.getStudentname(); } @Override public List<StudentDTO> getAllStudent() { List<Student> getStudent = studentRepo.findAll(); List<StudentDTO> studentDTOList = new ArrayList<>(); // for(Student student: getStudent) // { // StudentDTO studentDTO = new StudentDTO( // // student.getStudentid(), // student.getStudentname(), // student.getAddress(), // student.getMobile(), // student.isActive() // // ); // studentDTOList.add(studentDTO); List<StudentDTO> studentDTOS = modelMapper.map(getStudent, new TypeToken<List<StudentDTO>>(){}.getType()); return studentDTOS; } @Override public String updateStudent(StudentUpdateDTO studentUpdateDTO) { if(studentRepo.existsById(studentUpdateDTO.getStudentid())) { Student student = studentRepo.getById(studentUpdateDTO.getStudentid()); student.setStudentname(studentUpdateDTO.getStudentname()); student.setAddress(studentUpdateDTO.getAddress()); student.setMobile(studentUpdateDTO.getMobile()); student.setActive(studentUpdateDTO.isActive()); studentRepo.save(student); } else { System.out.println("Student ID not Exist"); } return null; } @Override public boolean deleteStudent(int id) { if(studentRepo.existsById(id)) { studentRepo.deleteById(id); } else { System.out.println("Student ID is Not Found"); } return false; } @Override public StudentDTO getStudentById(int id) { Optional<Student> student = studentRepo.findById(id); if(student.isPresent()) { // StudentDTO studentDTO = new StudentDTO( // student.get().getStudentid(), // student.get().getStudentname(), // student.get().getAddress(), // student.get().getMobile(), // student.get().isActive() // // ); // return studentDTO; StudentDTO studentDTO = modelMapper.map(student.get(),StudentDTO.class); return studentDTO; } else { System.out.println("Student ID Not Found"); } return null; } @Override public List<StudentDTO> findStudentName(String name) { List<Student> students = studentRepo.findAllByStudentnameEquals(name); if(students.size()!=0) { List<StudentDTO> studentDTOS = modelMapper.map(students,new TypeToken<List<StudentDTO>>(){}.getType()); return studentDTOS; } return null; } }
Building the Controller
Create the REST controller to handle HTTP requests:
package com.SpringProject.StudentCrud.controller; import com.SpringProject.StudentCrud.dto.StudentDTO; import com.SpringProject.StudentCrud.dto.StudentSaveDTO; import com.SpringProject.StudentCrud.dto.StudentUpdateDTO; import com.SpringProject.StudentCrud.service.StudentService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.crossstore.ChangeSetPersister; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @CrossOrigin(origins = "http://localhost:3000") @RequestMapping("api/v1/student") public class StudentController { @Autowired private StudentService studentService; @PostMapping(path = "/save") public String saveStudent(@RequestBody StudentSaveDTO studentSaveDTO) { String name = studentService.addStudent(studentSaveDTO); return "Record Addedd"; } @GetMapping("/getAllStudent") public List<StudentDTO> getAllStudent() { List<StudentDTO> allStudents = studentService.getAllStudent(); return allStudents; } @PutMapping("/update") public String updateStudent(@RequestBody StudentUpdateDTO studentUpdateDTO) { String id = studentService.updateStudent(studentUpdateDTO); return "updateddd"; } @DeleteMapping(path ="/delete/{id}") public String deleteStudent(@PathVariable(value="id")int id) { boolean deleteStudent = studentService.deleteStudent(id); return "deleted!!!!"; } @GetMapping( path = "/get-by-id", params = "id" ) public StudentDTO getStudentById(@RequestParam(value = "id")int id) { StudentDTO studentDTO = studentService.getStudentById(id); return studentDTO; } @GetMapping( path = "/get-by-name", params = "name" ) public List<StudentDTO> getStudentByName(@RequestParam(value = "name")String name) { List<StudentDTO> studentdetails = studentService.findStudentName(name); return studentdetails; } }
Frequently Asked Questions (FAQ)
What is CRUD in Spring Boot?
CRUD stands for Create, Read, Update, and Delete. In Spring Boot, these operations are handled through RESTful APIs and connected to databases like MySQL.
Why use Spring Boot with MySQL?
Spring Boot makes backend development easy with minimal configuration, while MySQL is a popular open-source relational database. Together, they provide a powerful tech stack.
Is the source code free to use?
Yes, the full source code is free to download and use in your own projects. It’s a great way to learn Spring Boot CRUD with MySQL by doing it yourself.
Can beginners follow this tutorial?
Absolutely. This tutorial is written in a beginner-friendly manner with step-by-step instructions to help you build a working REST API from scratch.
How do I run the project locally?
You’ll need to install Java, MySQL, and an IDE like IntelliJ or Eclipse. Clone the project, configure the database settings, and run it using Spring Boot.
Testing the API Endpoints
Use tools like Postman or curl to test the following endpoints:
- Create: POST /api/v1/students/save
- Read All: GET /api/v1/students/getAll
- Update: PUT /api/v1/students/update
- Delete: DELETE /api/v1/students/delete/{id}
- Read by ID: GET /api/v1/students/getById?id={id}
- Read by Name: GET /api/v1/students/getByName?name={name}
i have attached the video link below. which will do this tutorials step by step.