This tutorial will teach you Advanced Bus Booking System step by step.This system will use to manage the Bus Booking System.
The System shall be able to select the seat no which customer wants.
Step 1: implement MouseListener
public class pos extends javax.swing.JFrame implements MouseListener {
Step 2: set the addMouseListener(this) in to each label. write it inside the constructor.
lbl1.addMouseListener(this); lbl2.addMouseListener(this); lbl3.addMouseListener(this); lbl4.addMouseListener(this); lbl5.addMouseListener(this); lbl6.addMouseListener(this); lbl7.addMouseListener(this); lbl8.addMouseListener(this); lbl9.addMouseListener(this); lbl10.addMouseListener(this); lbl11.addMouseListener(this); lbl12.addMouseListener(this); Connect(); SimpleDateFormat formatter = new SimpleDateFormat("yyyy/MM/dd"); Date date = new Date(); txtdate.getDayChooser().setMinSelectableDate(date);
after implemented the MouseListener it ask to implement all the abstruct method of MouseListener.you must implement it.
after created all abstruct methods paste the code inside the mouseClicked method.
public void mouseClicked(MouseEvent e) { if(e.getSource() == lbl1 ) { seatno = 1; } else if(e.getSource() == lbl2 ) { seatno = 2; } else if(e.getSource() == lbl3 ) { seatno = 3; } else if(e.getSource() == lbl4 ) { seatno = 4; } else if(e.getSource() == lbl5 ) { seatno = 5; } else if(e.getSource() == lbl6 ) { seatno = 6; } else if(e.getSource() == lbl7 ) { seatno = 7; } else if(e.getSource() == lbl8 ) { seatno = 8; } else if(e.getSource() == lbl9 ) { seatno = 9; } else if(e.getSource() == lbl10 ) { seatno = 10; } else if(e.getSource() == lbl11 ) { seatno = 11; } else if(e.getSource() == lbl12 ) { seatno = 12; } } @Override public void mousePressed(MouseEvent e) { // throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } @Override public void mouseReleased(MouseEvent e) { // throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } @Override public void mouseEntered(MouseEvent e) { // throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } @Override public void mouseExited(MouseEvent e) { // throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
Estabilsh the database connection
Connection con; PreparedStatement pst; int seatno=0; ResultSet rs; public void Connect() { try { Class.forName("com.mysql.jdbc.Driver"); con = DriverManager.getConnection("jdbc:mysql://localhost/buss","root",""); } catch (ClassNotFoundException ex) { } catch (SQLException ex) { }
Paste code the inside the book button
String customer = txtcust.getText(); int seatno1 = seatno; String price = txtprice.getText(); SimpleDateFormat date_format = new SimpleDateFormat("yyyy-MM-dd"); String date = date_format.format(txtdate.getDate()); try { pst = con.prepareStatement("select * from book where date = ? and seatno = ?"); pst.setString(1, date); pst.setInt(2, seatno1); rs = pst.executeQuery(); if(rs.next() == true) { JOptionPane.showMessageDialog(this, "Sorry this has been Booked"); } else { try { pst = con.prepareStatement("insert into book(cname,seatno,price,date)values(?,?,?,?)"); pst.setString(1, customer); pst.setInt(2, seatno1); pst.setString(3, price); pst.setString(4, date); int k = pst.executeUpdate(); if(k==1) { JOptionPane.showMessageDialog(this, "Bus Booked"); bill(); } else { JOptionPane.showMessageDialog(this, "Record Failed"); } } catch (SQLException ex) { } } } catch (SQLException ex) { Logger.getLogger(busadvanc.class.getName()).log(Level.SEVERE, null, ex); }
Search Seats
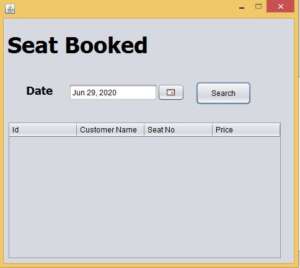
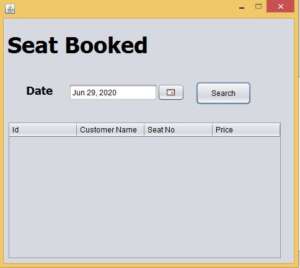
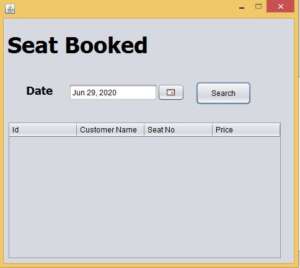
The System shall be to search the seats.
Connection con; PreparedStatement pst; ResultSet rs; int seatno=0; private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { SimpleDateFormat date_format = new SimpleDateFormat("yyyy-MM-dd"); String date1 = date_format.format(txtdate.getDate()); int c; try { pst = con.prepareStatement("select * from book where date = ?"); pst.setString(1, date1); rs = pst.executeQuery(); if(rs.next() == false) { JOptionPane.showMessageDialog(this, "Date Not Found"); } else { ResultSetMetaData rsd = rs.getMetaData(); c = rsd.getColumnCount(); DefaultTableModel d = (DefaultTableModel)jTable1.getModel(); d.setRowCount(0); while(rs.next()) { Vector v2 = new Vector(); for(int i= 1; i<=c; i++) { v2.add(rs.getString("id")); v2.add(rs.getString("cname")); v2.add(rs.getString("seatno")); v2.add(rs.getString("price")); } d.addRow(v2); } } } catch (SQLException ex) { Logger.getLogger(check.class.getName()).log(Level.SEVERE, null, ex); } }
i have attached the video link below. which will do this tutorials step by step.