In this tutorial will teach crud with Laravel. Laravel 8 CRUD Application we will cover about Create, Read, Update, and Delete and View crud operation in Laravel. Laravel is world best famous PHP framework.it has various features. Laravel is a MVC architecture. crud using Laravel example I will show in the simple way to make eloquent Laravel crud application. Here is the best place where learn Laravel 8.
I attached Laravel 8 eloquent crud example demo below screenshot.
What is crud in Laravel
CRUD stands for Create, Read, Update, and Delete which used to performed the database operations.
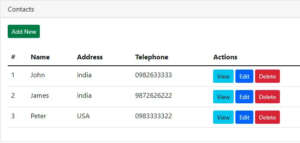
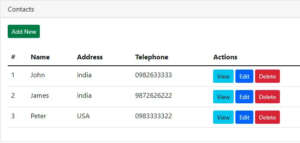
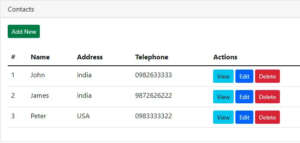
Lets do the Crud Step by Step Laravel 8 From Scratch
First Step
In computer create the folder Laravel Projects. And open the folder. and type the address bar on cmd
and Press Enter key and Open the Command Prompt.
Install Laravel 8
Create the new project which name is crudapplication
composer create-project --prefer-dist laravel/laravel crudapplication
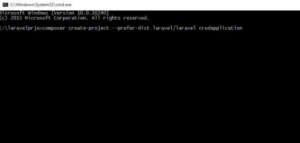
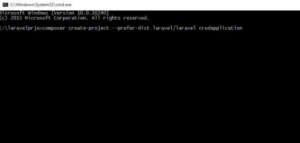
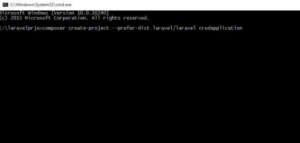
After Type the Command you have to wait till the project has been created. After that setup the database
Database setup
Create the Database on xampp which name is crud
After created the database.Do the database configuration on .env file which is reside on the Laravel folder structure
Change .env File
Do the database configuration on .env File for username, password and DB Name
if you install the lowerest version of Mysql do the following configatation i attached the screenshot image below.
Remove mb4 from Charset (config/database.php) // MySQL Version < 6
Run the Project
Run the Application using following command
php artisan serve
This is the Welcome Page of Laravel
Create Migrations
In Laravel create the table called as Migration
Run the command to create the Migration
php artisan make:migration create_contacts_table
After that you can check the inside database folder migrations create_contacts_table.php file has been created
successfully.
Select and open it
class CreateContactsTable extends Migration { public function up() { Schema::create('contacts', function (Blueprint $table) { $table->id(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('contacts'); } }
then create the following fields
$table->string(‘name’);
$table->string(‘address’);
$table->string(‘mobile’);
inside the function up() function shown in below clearly.
class CreateContactsTable extends Migration { public function up() { Schema::create('contacts', function (Blueprint $table) { $table->id(); $table->timestamps(); $table->string('name'); $table->string('address'); $table->string('mobile'); }); } public function down() { Schema::dropIfExists('contacts'); } }
After modified the columns names then run the migrate command to create the tables in the databases.before the run the
command please save project then run it.
php artisan migrate
After that your migration has been created successfully.will moving to the controller part of the project.
Create Controller
Create Controller
in order to create the controller if it crud you can use –resource controller.
what is Resource controller
This is used to implement the crud operation easy way. using the keyword –resource
Create the controller name which is ContactController after the controller name add it–resource
php artisan make:controller ContactController --resource
After create the controller need to create the model.
Create Model
Model is used to get the data from the database.
Create the Model name which is Crud
php artisan make:model Contact
After Model is Created the look like this. Code inside Model Class (app\Models\)
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Contact extends Model { use HasFactory; }
Add the table name and primarykey and columns names
protected $table = ‘contacts’;
protected $primaryKey = ‘id’;
protected $fillable = [‘name’, ‘address’, ‘mobile’];
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Contact extends Model { protected $table = 'contacts'; protected $primaryKey = 'id'; protected $fillable = ['name', 'address', 'mobile']; }
Create Views
Create a Folder inside the resources-views
inside the views folder create the contacts folder inside the folder create the following pages
- layout.blade.php
- index.blade.php
- Create.blade.php
- edit.blade.php
- show.blade.php
In Laravel you have create the pages using pagename.blade.php.
Create page which is layoutpage
layout.blade.php
<!DOCTYPE html> <html> <head> <title>Contact Laravel 8 CRUD</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous"> </head> <body> <div class="container"> @yield('content') </div> </body> </html>
Create page index.blade.php
@extends('contacts.layout') @section('content') <div class="container"> <div class="row"> <div class="col-md-9"> <div class="card"> <div class="card-header">Contacts</div> <div class="card-body"> <a href="{{ url('/contact/create') }}" class="btn btn-success btn-sm" title="Add New Contact"> <i class="fa fa-plus" aria-hidden="true"></i> Add New </a> <br/> <br/> <div class="table-responsive"> <table class="table"> <thead> <tr> <th>#</th> <th>Name</th> <th>Address</th> <th>Telephone</th> <th>Actions</th> </tr> </thead> <tbody> @foreach($contacts as $item) <tr> <td>{{ $loop->iteration }}</td> <td>{{ $item->name }}</td> <td>{{ $item->address }}</td> <td>{{ $item->mobile }}</td> <td> <a href="{{ url('/contact/' . $item->id) }}" title="View Student"><button class="btn btn-info btn-sm"><i class="fa fa-eye" aria-hidden="true"></i> View</button></a> <a href="{{ url('/contact/' . $item->id . '/edit') }}" title="Edit Student"><button class="btn btn-primary btn-sm"><i class="fa fa-pencil-square-o" aria-hidden="true"></i> Edit</button></a> <form method="POST" action="{{ url('/contact' . '/' . $item->id) }}" accept-charset="UTF-8" style="display:inline"> {{ method_field('DELETE') }} {{ csrf_field() }} <button type="submit" class="btn btn-danger btn-sm" title="Delete Contact" onclick="return confirm("Confirm delete?")"><i class="fa fa-trash-o" aria-hidden="true"></i> Delete</button> </form> </td> </tr> @endforeach </tbody> </table> </div> </div> </div> </div> </div> </div> @endsection
Add Records
Create page Create.blade.php
@extends('contacts.layout') @section('content') <div class="card"> <div class="card-header">Contactus Page</div> <div class="card-body"> <form action="{{ url('contact') }}" method="post"> {!! csrf_field() !!} <label>Name</label></br> <input type="text" name="name" id="name" class="form-control"></br> <label>Address</label></br> <input type="text" name="address" id="address" class="form-control"></br> <label>Mobile</label></br> <input type="text" name="mobile" id="mobile" class="form-control"></br> <input type="submit" value="Save" class="btn btn-success"></br> </form> </div> </div> @stop
Edit Records
Create page edit.blade.php
@extends('contacts.layout') @section('content') <div class="card"> <div class="card-header">Contactus Page</div> <div class="card-body"> <form action="{{ url('contact/' .$contacts->id) }}" method="post"> {!! csrf_field() !!} @method("PATCH") <input type="hidden" name="id" id="id" value="{{$contacts->id}}" id="id" /> <label>Name</label></br> <input type="text" name="name" id="name" value="{{$contacts->name}}" class="form-control"></br> <label>Address</label></br> <input type="text" name="address" id="address" value="{{$contacts->address}}" class="form-control"></br> <label>Mobile</label></br> <input type="text" name="mobile" id="mobile" value="{{$contacts->mobile}}" class="form-control"></br> <input type="submit" value="Update" class="btn btn-success"></br> </form> </div> </div> @stop
Show Records
Create page show.blade.php
@extends('contacts.layout') @section('content') <div class="card"> <div class="card-header">Contactus Page</div> <div class="card-body"> <div class="card-body"> <h5 class="card-title">Name : {{ $contacts->name }}</h5> <p class="card-text">Address : {{ $contacts->address }}</p> <p class="card-text">Phone : {{ $contacts->mobile }}</p> </div> </hr> </div> </div>
Controller
After that Pass All view pages through Controller. you have to add the Model namespace here
use App\Models\Contact; Data is coming from the database via Model.
<?php namespace App\Http\Controllers; use App\Models\Contact; use Illuminate\Http\Request; class ContactController extends Controller { public function index() { $contacts = Contact::all(); return view ('contacts.index')->with('contacts', $contacts); } public function create() { return view('contacts.create'); } public function store(Request $request) { $input = $request->all(); Contact::create($input); return redirect('contact')->with('flash_message', 'Contact Addedd!'); } public function show($id) { $contact = Contact::find($id); return view('contacts.show')->with('contacts', $contact); } public function edit($id) { $contact = Contact::find($id); return view('contacts.edit')->with('contacts', $contact); } public function update(Request $request, $id) { $contact = Contact::find($id); $input = $request->all(); $contact->update($input); return redirect('contact')->with('flash_message', 'Contact Updated!'); } public function destroy($id) { Contact::destroy($id); return redirect('contact')->with('flash_message', 'Contact deleted!'); } }
Routes
Pages are Manage through routes. If you are crud system simple you can add it the routes one line look like this
Route::resource(‘/contact’, ContactController::class);
You have to add the ControllerNameSpace
use App\Http\Controllers\ContactController;
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ContactController; Route::get('/', function () { return view('welcome'); }); Route::resource('/contact', ContactController::class);
Laravel 8 Projects
How to Make a Laravel website with contact form
https://www.tutussfunny.com/basic-laravel-8-project-step-by-step/
Laravel 8 Join Two Tables
https://www.tutussfunny.com/laravel-8-join-two-tables/
Laravel 8 Crud using crud generator tool with Authentication
Easy way to implement the crud using crud generator tool(CREATE,READ,UPDATE,DELETE)
https://youtu.be/ZP8QKQMZUxk
i have attached the video link below. which will do this tutorials step by step.