This Laravel 9 Crud Application will help you learn basic crud operation are create,read,delete,update.Laravel is world famous Number one php framework.it has various features.Laravel is a MVC architecture. In this tutorial will see how to make a crud application in Laravel 9.Laravel 9 – CRUD application tutorial with Example. Here is the Best Place to Learn Laravel 9 Articles.
Lets do the Crud Step by Step Laravel 9 From Scratch
First Step
Inside the project Folder Address Bar type cmd
and Press Enter key and Open the Command Prompt.
Install Laravel 9
Create a new Project type the command on the command prompt . I create the project name crudapplication
Laravel Tutorial Setup the Project
composer create-project --prefer-dist laravel/laravel crudapplication
After Type the Command you have to wait till the project has been created.
Database setup
Create the Database on xampp which name is basiccrud
After created the database.
Change .env File
Change .env File for username, password and DB Name
After that run check the application the welcome screen of Laravel framework look like this.
After that run check the application the welcome screen of Laravel framework look like this.
php artisan serve
Create Migration
Create the tables
php artisan make:migration create_students_table
After that you can check the inside database folder migrations create_students_table.php
Click and open the Student table.
public function up() { Schema::create('students', function (Blueprint $table) { $table->id(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('students'); }
then create the following fields
$table->string(‘name’);
$table->string(‘address’);
$table->string(‘mobile’);
inside the function up() function i shown in below clearly.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { public function up() { Schema::create('students', function (Blueprint $table) { $table->id(); $table->string("name"); $table->string("address"); $table->string("mobile"); $table->timestamps(); }); } public function down() { Schema::dropIfExists('students'); } };
After add the lines type
php artisan migrate
then table has been migrated
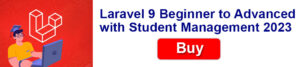
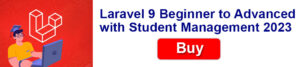
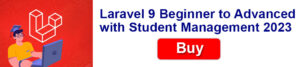
Create Controller
Create the controller name which is StudentController
php artisan make:controller StudentController --resource
Controller
After that Pass All view pages through Controller. you have to add the Model namespace here
use App\Models\Student; Data is coming from the database via Model.
Student Controller
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class StudentController extends Controller { public function index() { $students = Student::all(); return view ('students.index')->with('students', $students); } public function create() { return view('students.create'); } public function store(Request $request) { $input = $request->all(); Student::create($input); return redirect('student')->with('flash_message', 'Student Addedd!'); } public function show($id) { $student = Student::find($id); return view('students.show')->with('students', $student); } public function edit($id) { $student = Student::find($id); return view('students.edit')->with('students', $student); } public function update(Request $request, $id) { $student = Student::find($id); $input = $request->all(); $student->update($input); return redirect('student')->with('flash_message', 'student Updated!'); } public function destroy($id) { Student::destroy($id); return redirect('student')->with('flash_message', 'Student deleted!'); } }
Create Model
Model is used to get the data from the database.
Create the Model name which is Student
php artisan make:model Student
After Model is Created the look like this. Code inside Model Class (app\Models\)
Change it as like this
Add the Namespace above
namespace App\Models;
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Student extends Model { protected $table = 'students'; protected $primaryKey = 'id'; protected $fillable = ['name', 'address', 'mobile']; }
Create Views
Create a Folder inside the resources-views
inside the views folder create the students folder
In Laravel you have create the pages using pagename.blade.php
Create pages
layout.blade.php
<!DOCTYPE html> <html> <head> <title>Student Laravel 9 CRUD</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous"> </head> <body> <div class="container"> @yield('content') </div> </body> </html>
index.blade.php
@extends('students.layout') @section('content') <div class="container"> <div class="row"> <div class="col-md-9"> <div class="card"> <div class="card-header"> <h2>Laravel 9 Crud</h2> </div> <div class="card-body"> <a href="{{ url('/student/create') }}" class="btn btn-success btn-sm" title="Add New Student"> <i class="fa fa-plus" aria-hidden="true"></i> Add New </a> <br/> <br/> <div class="table-responsive"> <table class="table"> <thead> <tr> <th>#</th> <th>Name</th> <th>Address</th> <th>Mobile</th> <th>Actions</th> </tr> </thead> <tbody> @foreach($students as $item) <tr> <td>{{ $loop->iteration }}</td> <td>{{ $item->name }}</td> <td>{{ $item->address }}</td> <td>{{ $item->mobile }}</td> <td> <a href="{{ url('/student/' . $item->id) }}" title="View Student"><button class="btn btn-info btn-sm"><i class="fa fa-eye" aria-hidden="true"></i> View</button></a> <a href="{{ url('/student/' . $item->id . '/edit') }}" title="Edit Student"><button class="btn btn-primary btn-sm"><i class="fa fa-pencil-square-o" aria-hidden="true"></i> Edit</button></a> <form method="POST" action="{{ url('/student' . '/' . $item->id) }}" accept-charset="UTF-8" style="display:inline"> {{ method_field('DELETE') }} {{ csrf_field() }} <button type="submit" class="btn btn-danger btn-sm" title="Delete Student" onclick="return confirm("Confirm delete?")"><i class="fa fa-trash-o" aria-hidden="true"></i> Delete</button> </form> </td> </tr> @endforeach </tbody> </table> </div> </div> </div> </div> </div> </div> @endsection
create.blade.php
@extends('students.layout') @section('content') <div class="card"> <div class="card-header">Students Page</div> <div class="card-body"> <form action="{{ url('student') }}" method="post"> {!! csrf_field() !!} <label>Name</label></br> <input type="text" name="name" id="name" class="form-control"></br> <label>Address</label></br> <input type="text" name="address" id="address" class="form-control"></br> <label>Mobile</label></br> <input type="text" name="mobile" id="mobile" class="form-control"></br> <input type="submit" value="Save" class="btn btn-success"></br> </form> </div> </div> @stop
edit.blade.php
@extends('students.layout') @section('content') <div class="card"> <div class="card-header">Contactus Page</div> <div class="card-body"> <form action="{{ url('student/' .$students->id) }}" method="post"> {!! csrf_field() !!} @method("PATCH") <input type="hidden" name="id" id="id" value="{{$students->id}}" id="id" /> <label>Name</label></br> <input type="text" name="name" id="name" value="{{$students->name}}" class="form-control"></br> <label>Address</label></br> <input type="text" name="address" id="address" value="{{$students->address}}" class="form-control"></br> <label>Mobile</label></br> <input type="text" name="mobile" id="mobile" value="{{$students->mobile}}" class="form-control"></br> <input type="submit" value="Update" class="btn btn-success"></br> </form> </div> </div> @stop
show.blade.php
@extends('students.layout') @section('content') <div class="card"> <div class="card-header">Students Page</div> <div class="card-body"> <div class="card-body"> <h5 class="card-title">Name : {{ $students->name }}</h5> <p class="card-text">Address : {{ $students->address }}</p> <p class="card-text">Mobile : {{ $students->mobile }}</p> </div> </hr> </div> </div>
Routes
Pages are Manage through routes. If you are crud system simple you can add it the routes one line look like this
Route::resource(‘/contact’, StudentController::class);
You have to add the ControllerNameSpace
use App\Http\Controllers\StudentController;
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\StudentController; Route::get('/', function () { return view('welcome'); }); Route::resource("/student", StudentController::class);